Tokenizing a Gift Card
To tokenize a gift card for a gift card program supported by your site, you can make use of our tokenize gift card API's. Unlike our scheme card tokenization service which necessitates the use of our PCI compliant Frames SDK , for gift cards you can host your own frames to capture the gift card number
and pin
.
High-level flow
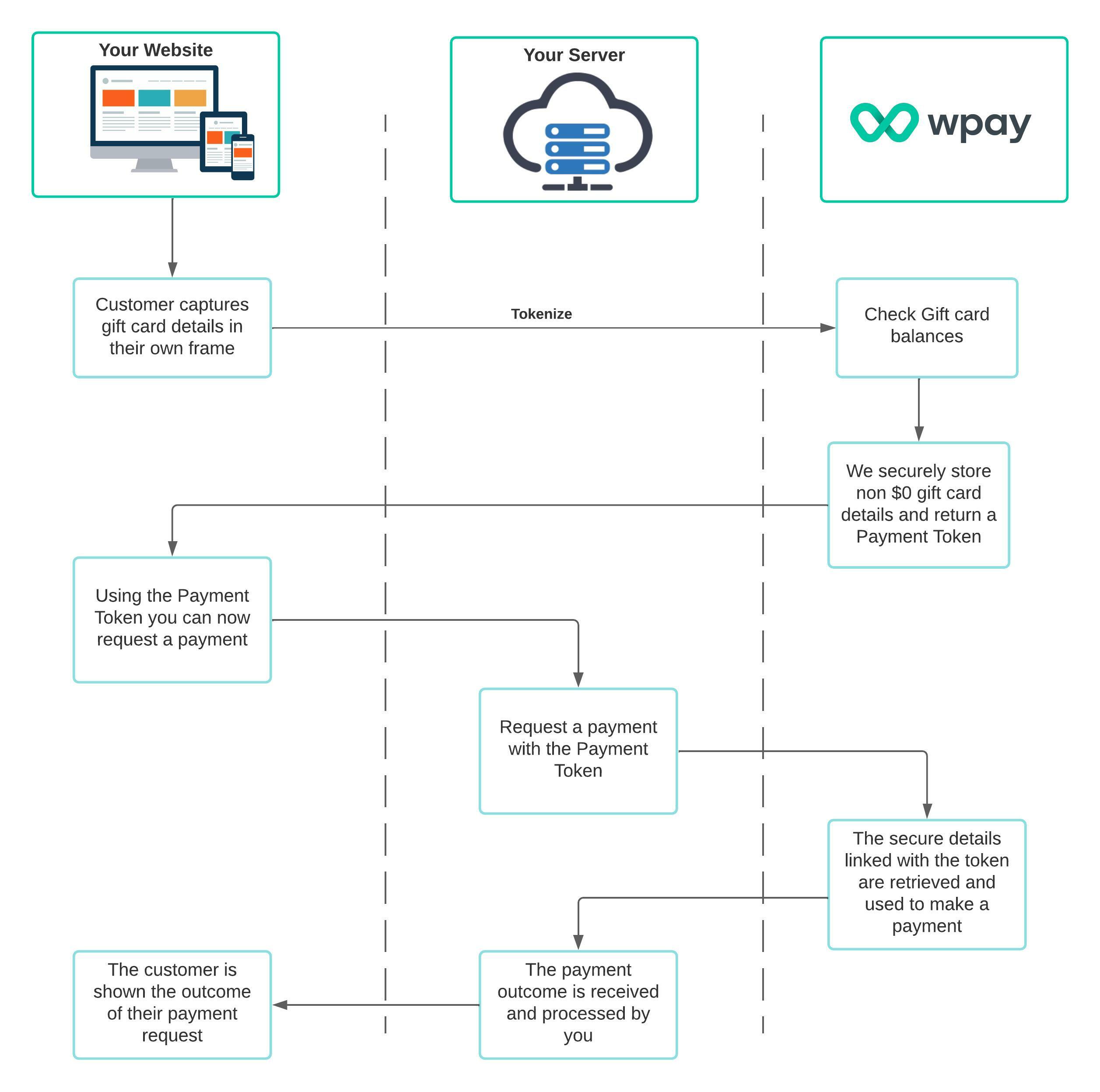
How it works
- Create and host frames on your site allowing your customers to capture a
gift card number
andpin
for a supported gift card program - Your customer's gift card information is processed by us and we return a
payment token
which is a unique representation of the gift card without any sensitive information. - The
payment token
can be used in our payments services to make a payment
Tokenizing a Gift Card
This method should be used to tokenize gift cards. The same API can be used for registered and guest customers.
curl --location --request POST 'https://{{environment}}.wpay.com.au/v1/apm/tokenize' \
--header 'Content-Type: application/json' \
--header 'X-Api-Key: {{yourApiKey}}' \
--header 'Authorization: Bearer {{yourBearerToken}}' \
--data-raw '{
"data": {
"paymentInstrumentType": "GIFTCARD",
"payload": {
"cardNumber": "628000*************",
"pinCode": "****",
"primary": false,
"save": true
}
},
"meta": {}
}'
var myHeaders = new Headers();
var environment = "substitute environment-value here"
var yourAPIkey = "YOUR-API-KEY";
var accessToken = "ACCESS-TOKEN";
myHeaders.append("Content-Type", "application/json");
myHeaders.append("X-Api-Key", yourAPIkey);
myHeaders.append("Authorization", `Bearer ${accessToken}`);
var raw = JSON.stringify({
"data": {
"paymentInstrumentType": "GIFTCARD",
"payload": {
"cardNumber": "628000*************",
"pinCode": "****",
"primary": false,
"save": true
}
},
"meta": {}
});
var requestOptions = {
method: 'POST',
headers: myHeaders,
body: raw,
redirect: 'follow'
};
fetch(`https://${environment}.wpay.com.au/wow/v1/pay/giftcards/tokenize`, requestOptions)
.then(response => response.text())
.then(result => console.log(result))
.catch(error => console.log('error', error));
import Foundation
#if canImport(FoundationNetworking)
import FoundationNetworking
#endif
var semaphore = DispatchSemaphore (value: 0)
let yourAPIkey = "YOUR-API-KEY";
let environment = "substitute environment-value here"
let accessToken = "ACCESS-TOKEN";
let parameters = """
{\
\"data\": {\
\"paymentInstrumentType\": \"GIFTCARD\",\
\"payload\": {\
\"cardNumber\": \"628000*************\",\
\"pinCode\": \"****\",\
\"primary\": false,\
\"save\": true\
}\
},\
\"meta\": {}\
}\
"""
let postData = parameters.data(using: .utf8)
var request = URLRequest(
url: URL(string: "https://\(environment).wpay.com.au/wow/v1/pay/giftcards/tokenize")!,
timeoutInterval: Double.infinity
)
request.addValue("application/json", forHTTPHeaderField: "Content-Type")
request.addValue(yourAPIkey, forHTTPHeaderField: "X-Api-Key")
request.addValue("Bearer \(accessToken)", forHTTPHeaderField: "Authorization")
request.httpMethod = "POST"
request.httpBody = postData
let task = URLSession.shared.dataTask(with: request) { data, response, error in
guard let data = data else {
print(String(describing: error))
semaphore.signal()
return
}
print(String(data: data, encoding: .utf8)!)
semaphore.signal()
}
task.resume()
semaphore.wait()
var environment = "substitute environment-value here"
var yourAPIkey = "YOUR-API-KEY"
var accessToken = "ACCESS-TOKEN"
val response = khttp.post(
url = "https://$environment" +
".wpay.com.au/wow/v1/pay/giftcards/tokenize",
headers = mapOf("Content-Type" to "application/json",
"X-Api-Key" to yourAPIkey,
"Authorization", "Bearer $accessToken"),
json = mapOf("data" to mapOf (
"paymentInstrumentType" to "APPLEPAY",
"payload" to mapOf (
"cardNumber" to "628000*************",
"pinCode" to "****",
"primary" to false,
"save" to true
)
),
"meta" to mapOf ()
)
)
if(response.statusCode == 200) {
val obj : JSONObject = response.jsonObject
println("Successful response payload: ${obj}")
} else {
handleError(response)
}
Where:
cardNumber
andpinCode
are the values for the gift card your customer wishes to tokenizeprimary
can be set totrue
orfalse
and this indicates whether it is a primary or secondary instrument when saving the card to the customer's walletsave
can be set totrue
orfalse
and this indicates whether to save the card in the customer's wallet after tokenizing (multi-use token) or to only have a token be valid for a single transaction (single-use token)
Updated about 2 years ago